Serving Data for Production AI
Feast is an open source feature store that delivers structured data to AI and LLM applications at high scale during training and inference
[ADOPTERS AND CONTRIBUTORS]

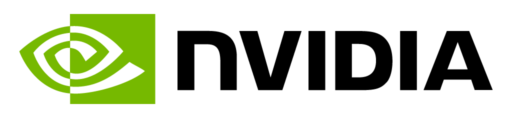
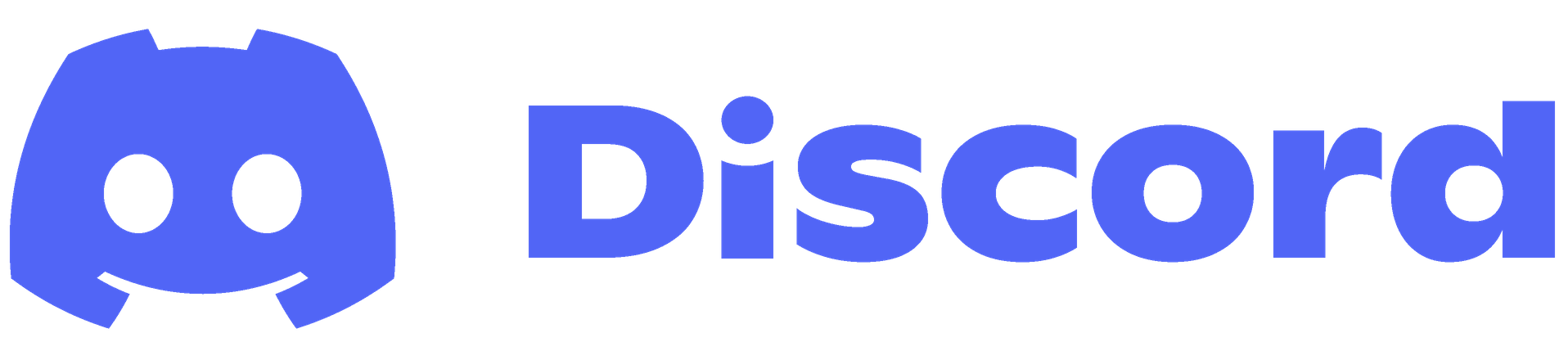
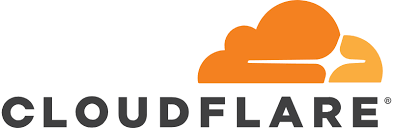
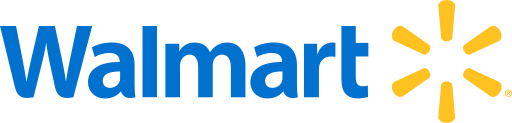
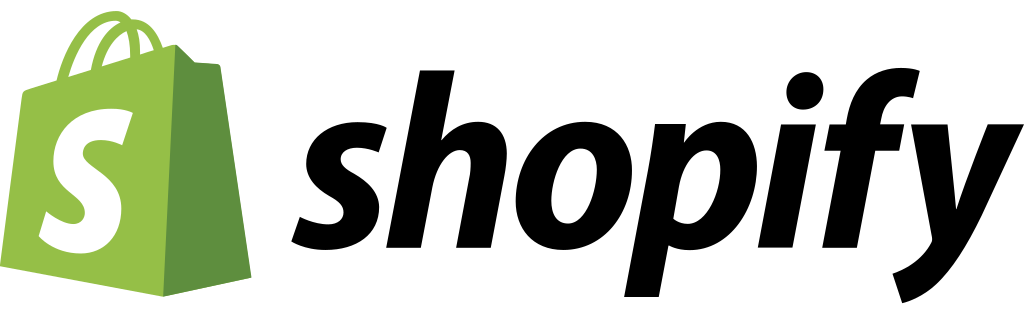
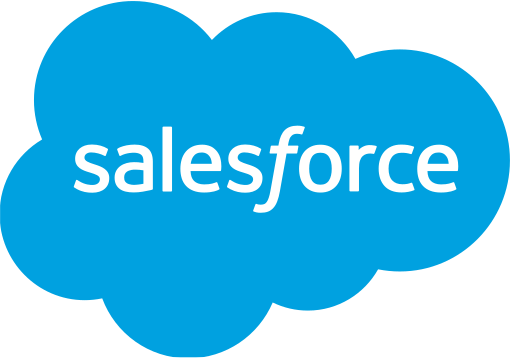
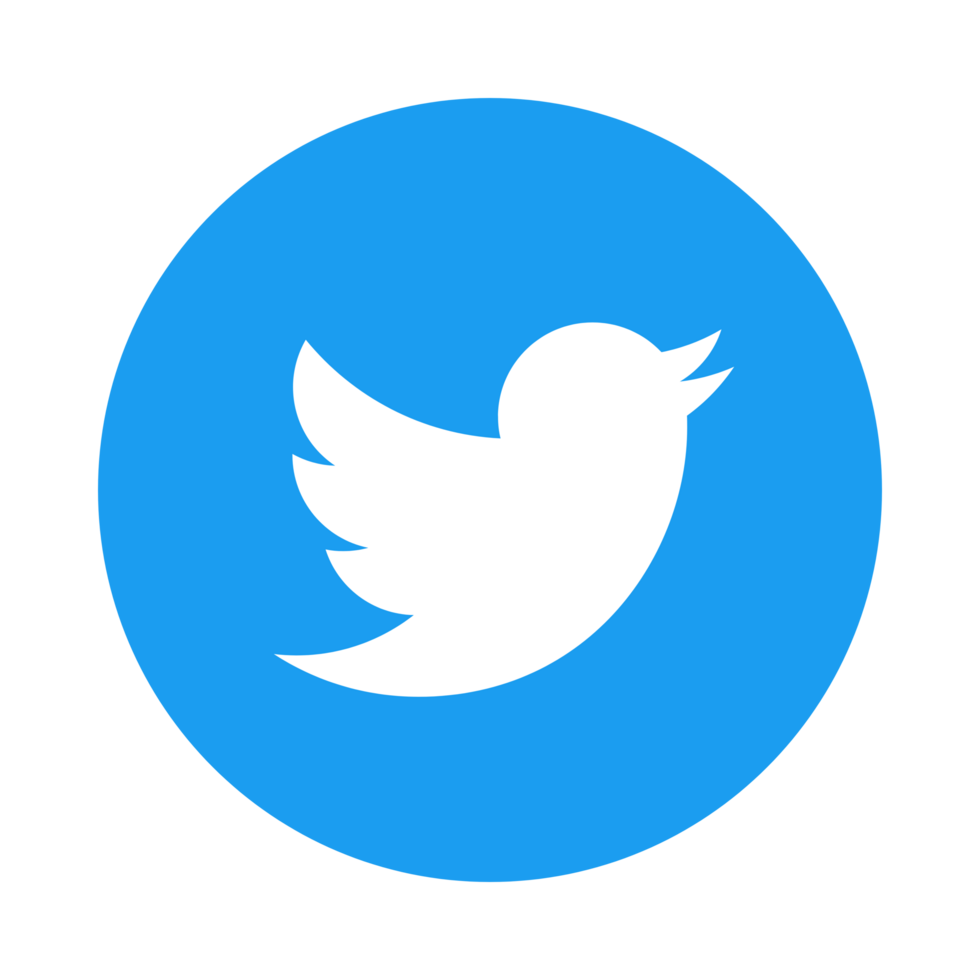
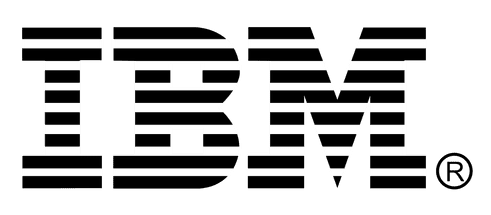
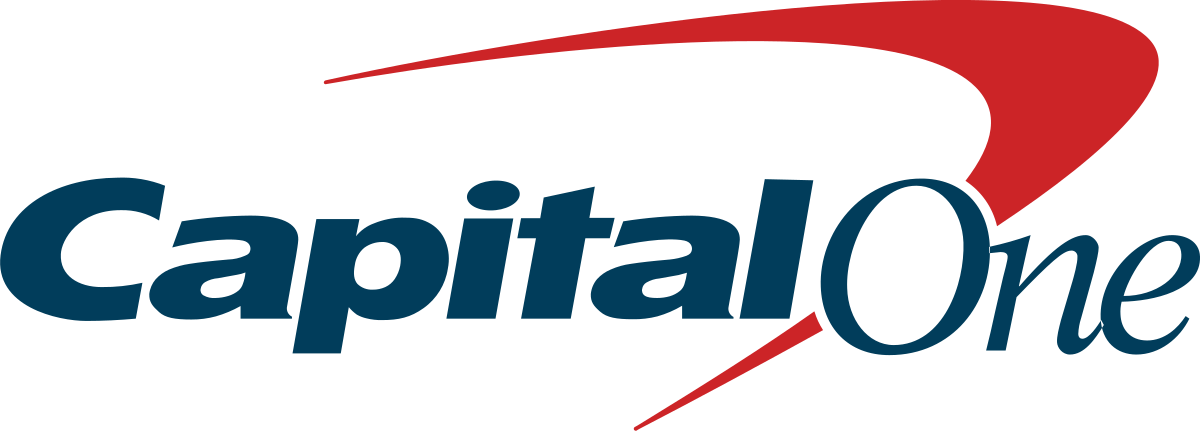
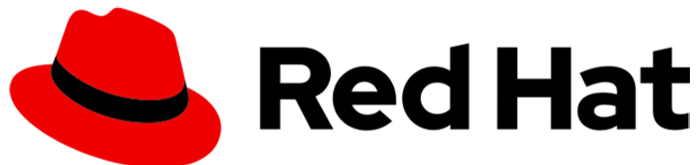
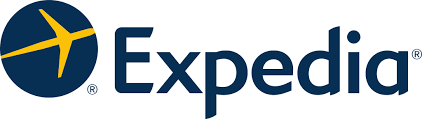
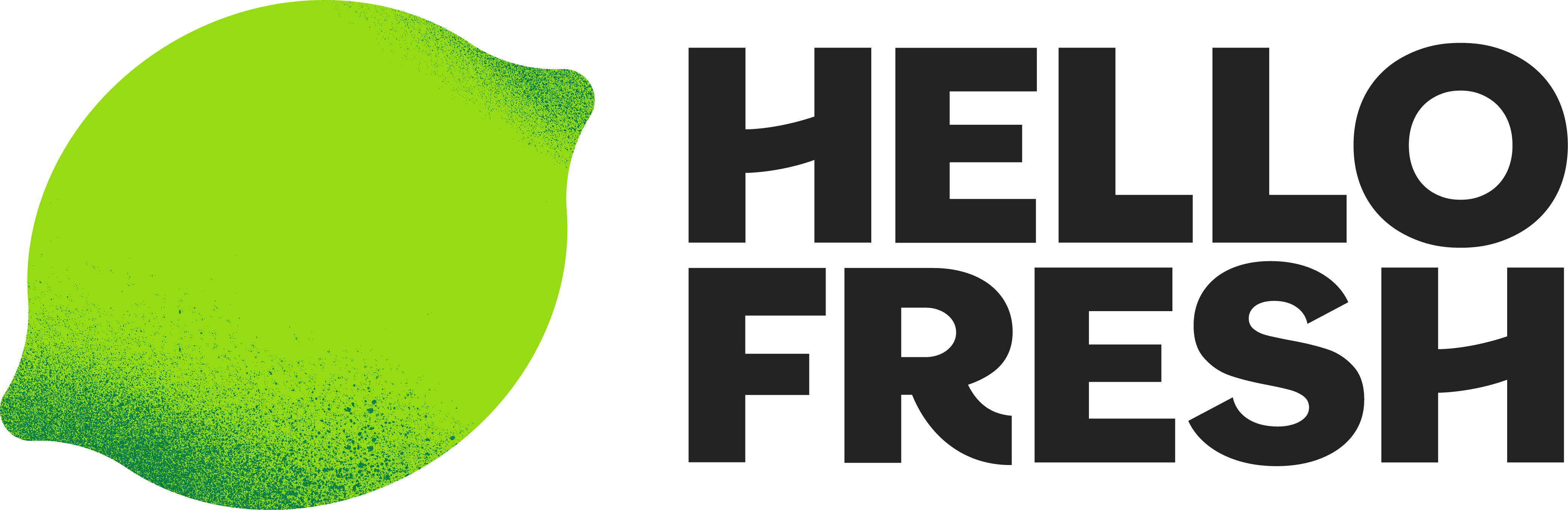
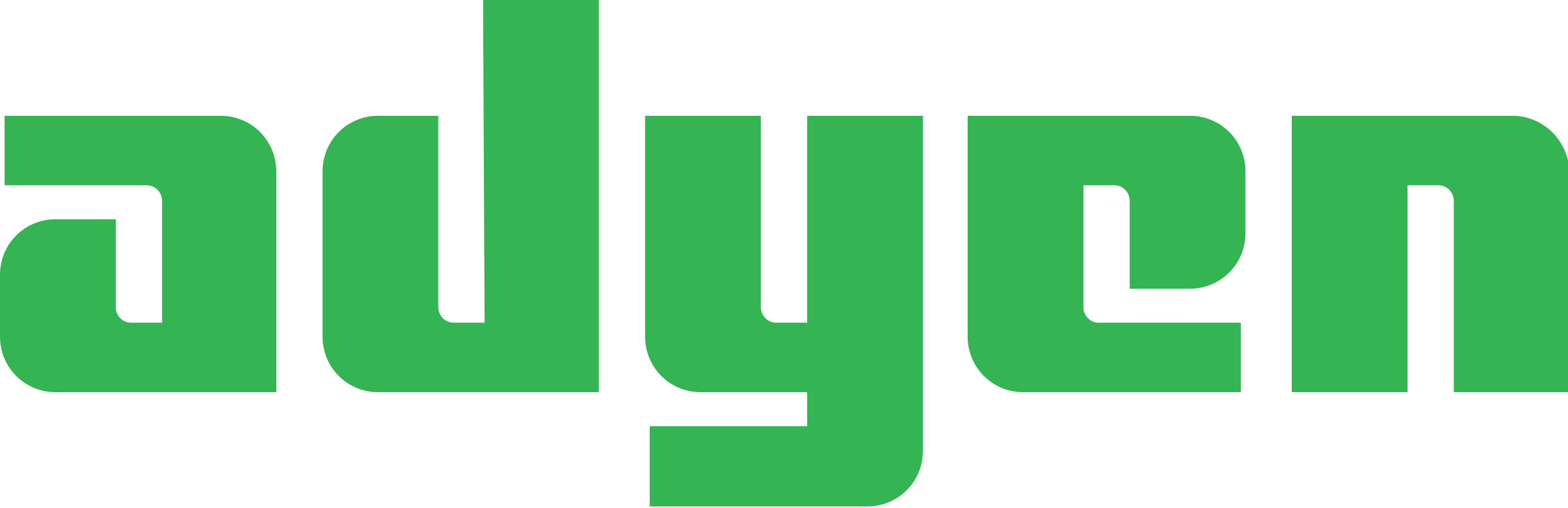
[USE CASES]
SOLVE REAL PROBLEMS
Real-Time Recommendations
Serve personalized product and content recommendations with real-time user interaction features
Fraud Detection
Detect fraudulent transactions using historical patterns and real-time behavioral features
Risk Scoring
Calculate risk scores for financial services using consistent features across training and inference
Customer Segmentation
Create dynamic customer segments using consistent feature definitions across teams
[INTEGRATIONS]
CONNECT WITH YOUR STACK
OFFLINE STORES
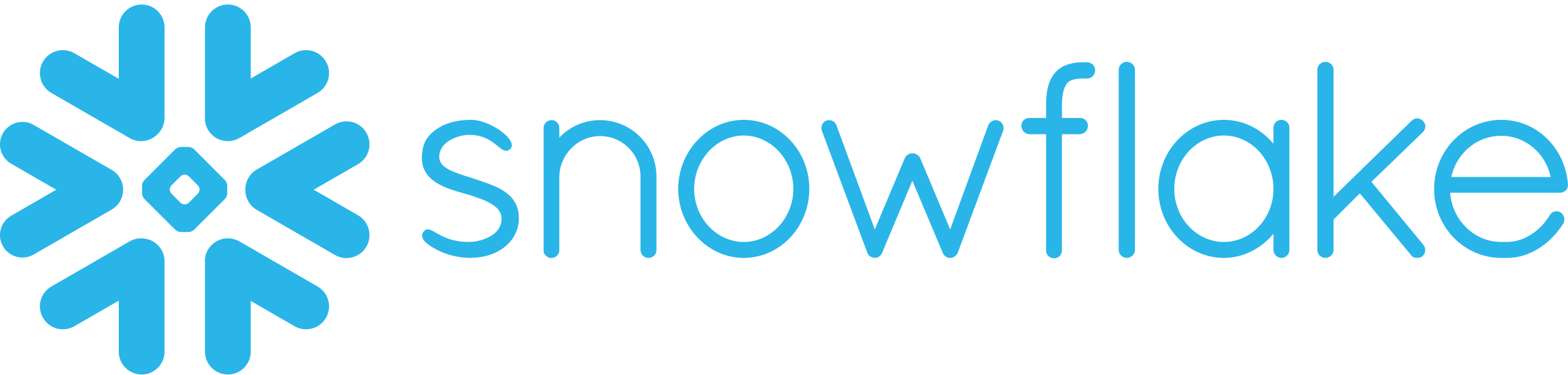
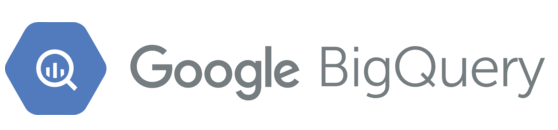
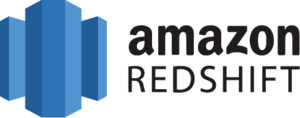
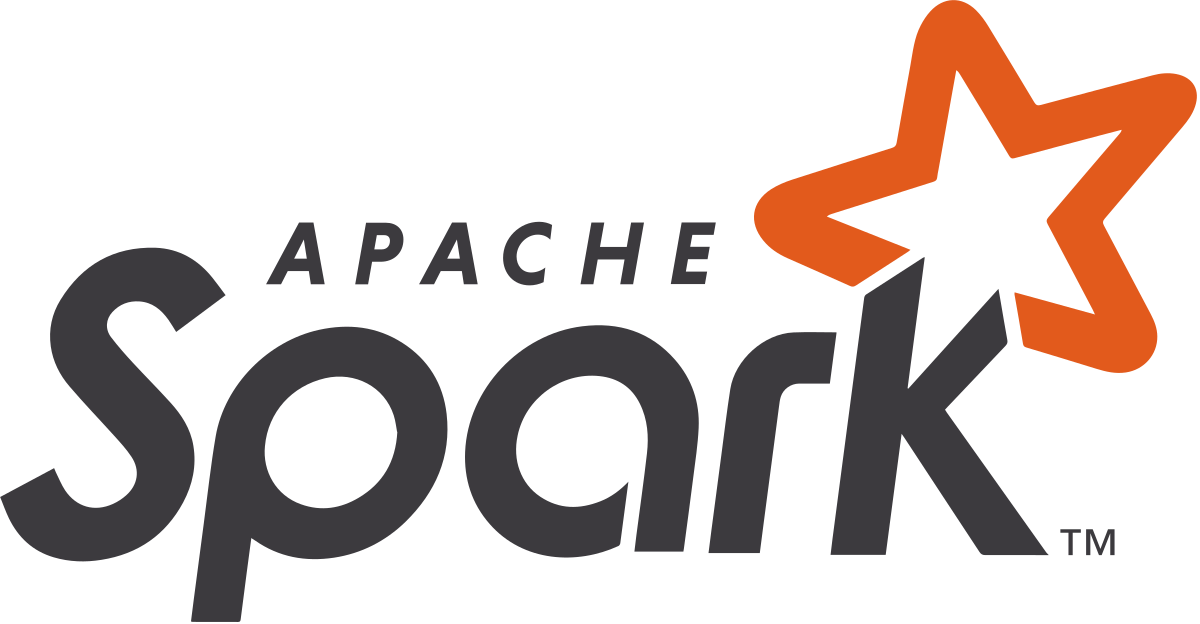
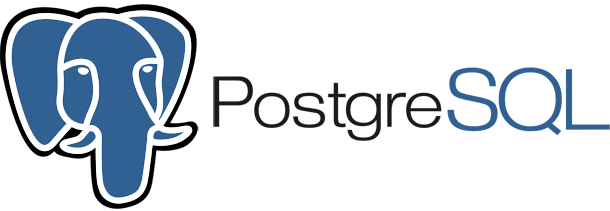
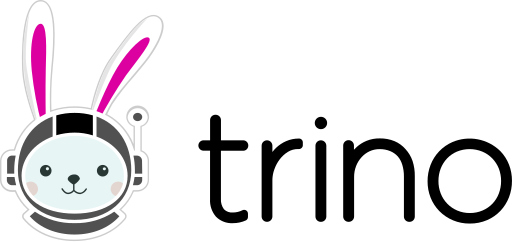
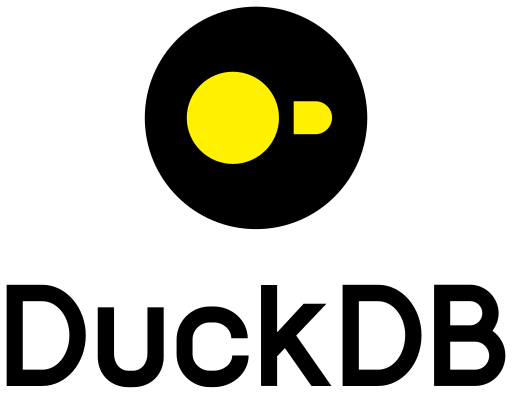
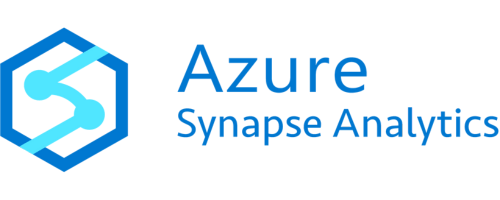
ONLINE STORES
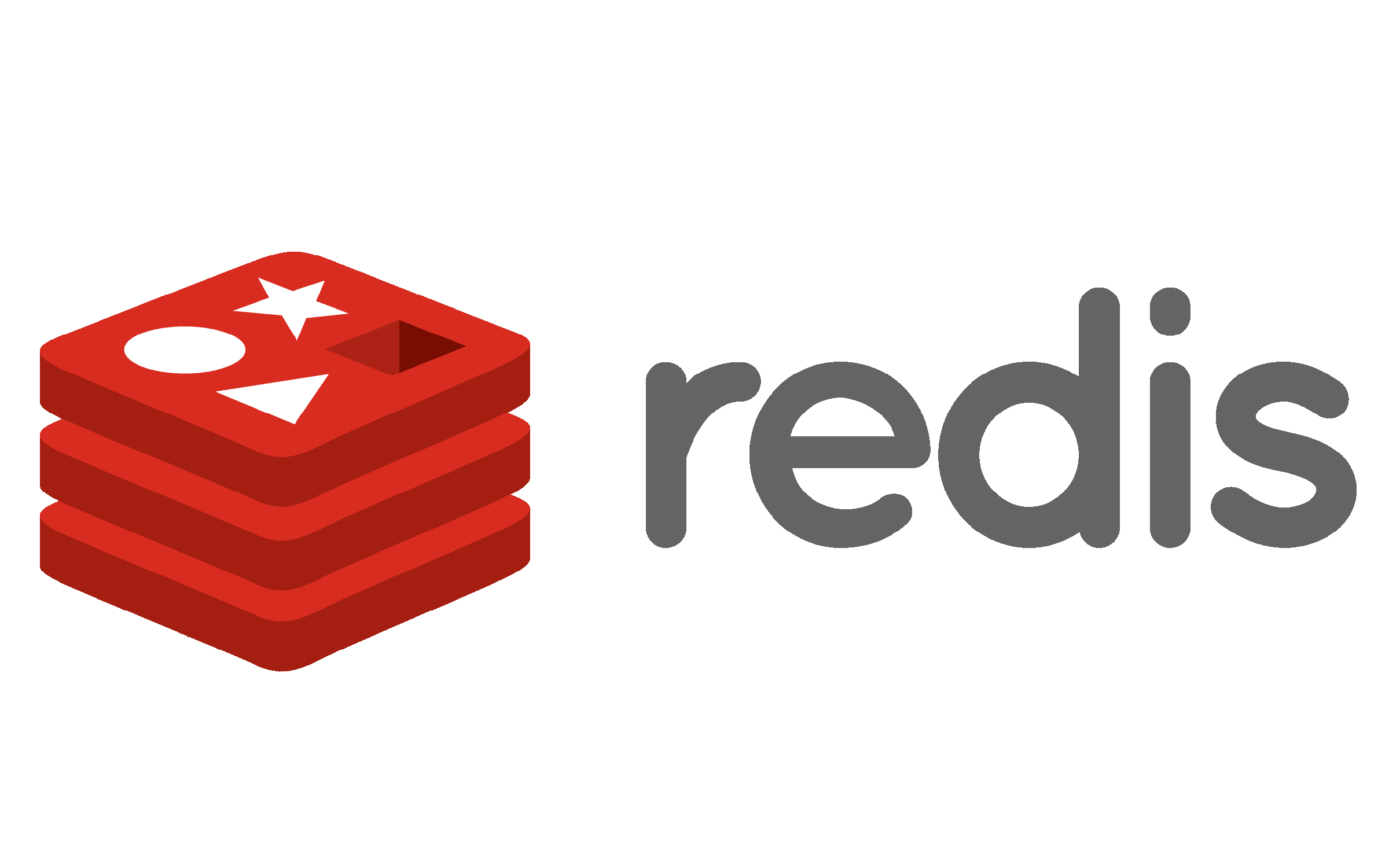
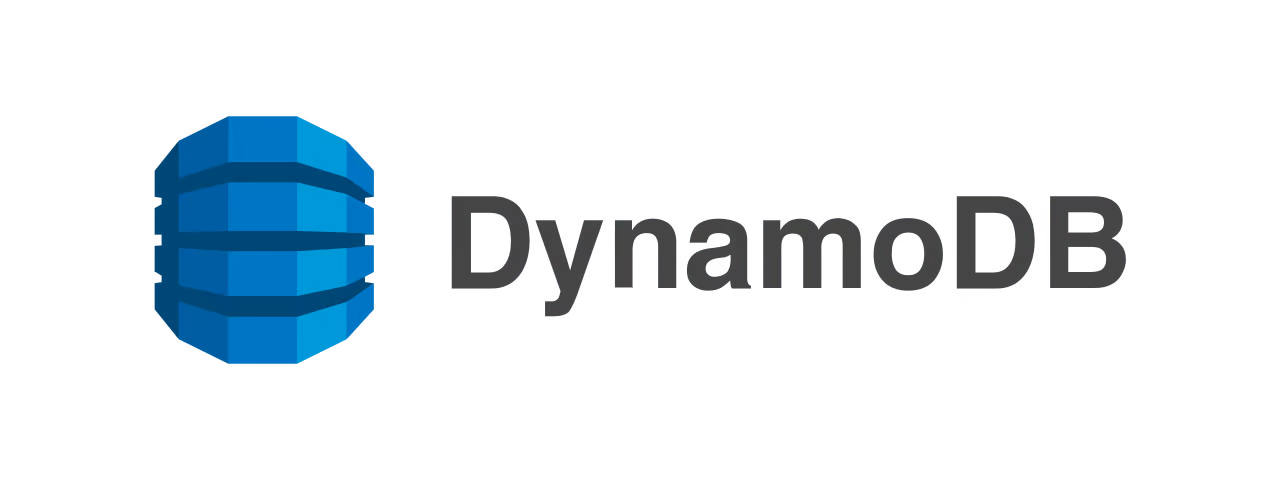
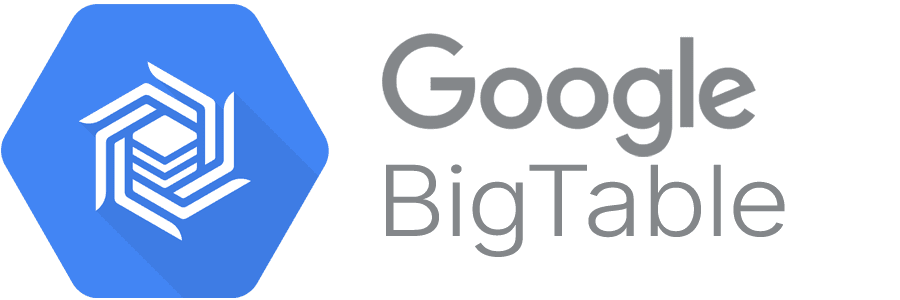
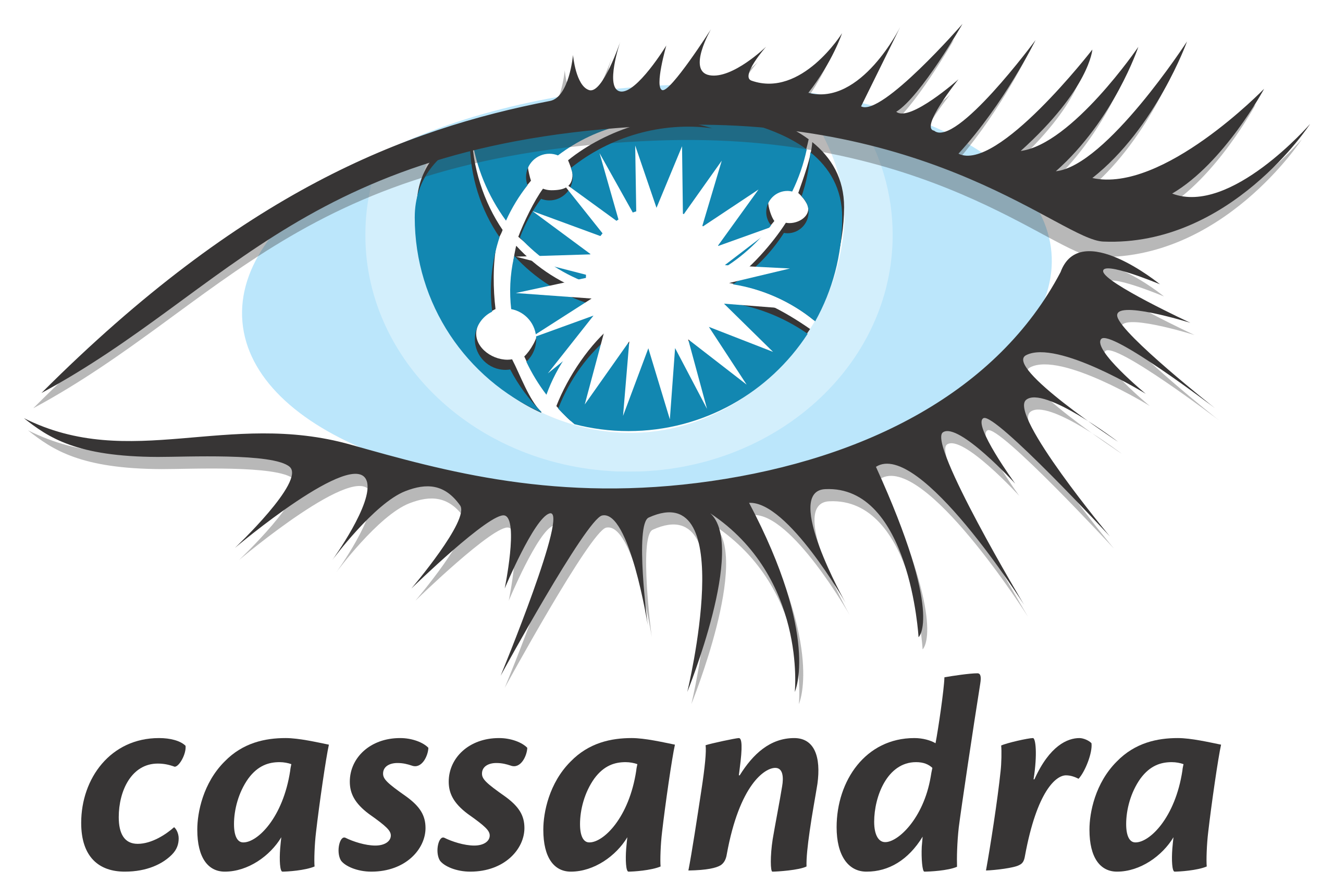
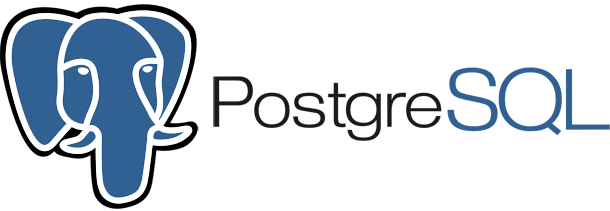
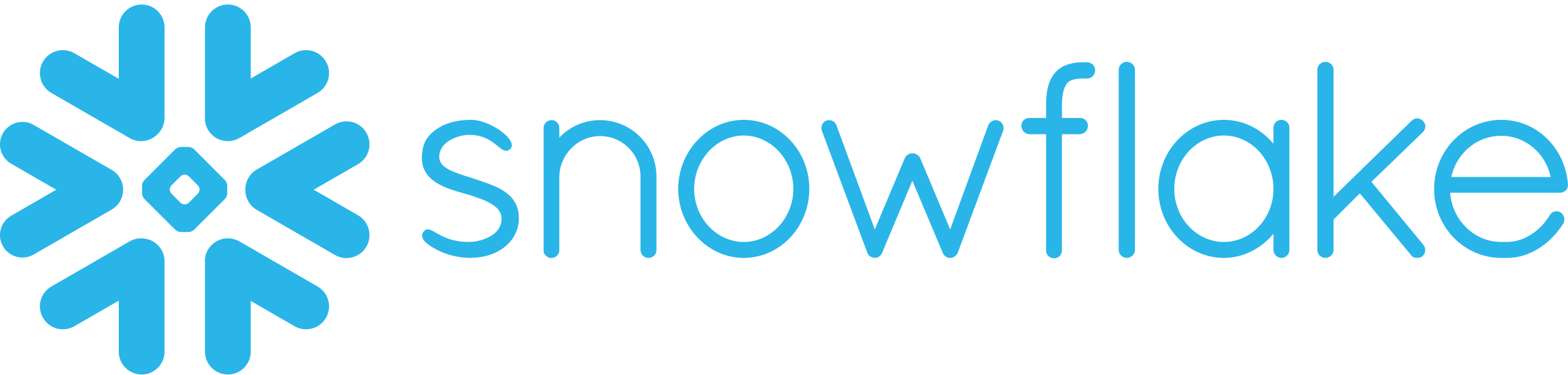
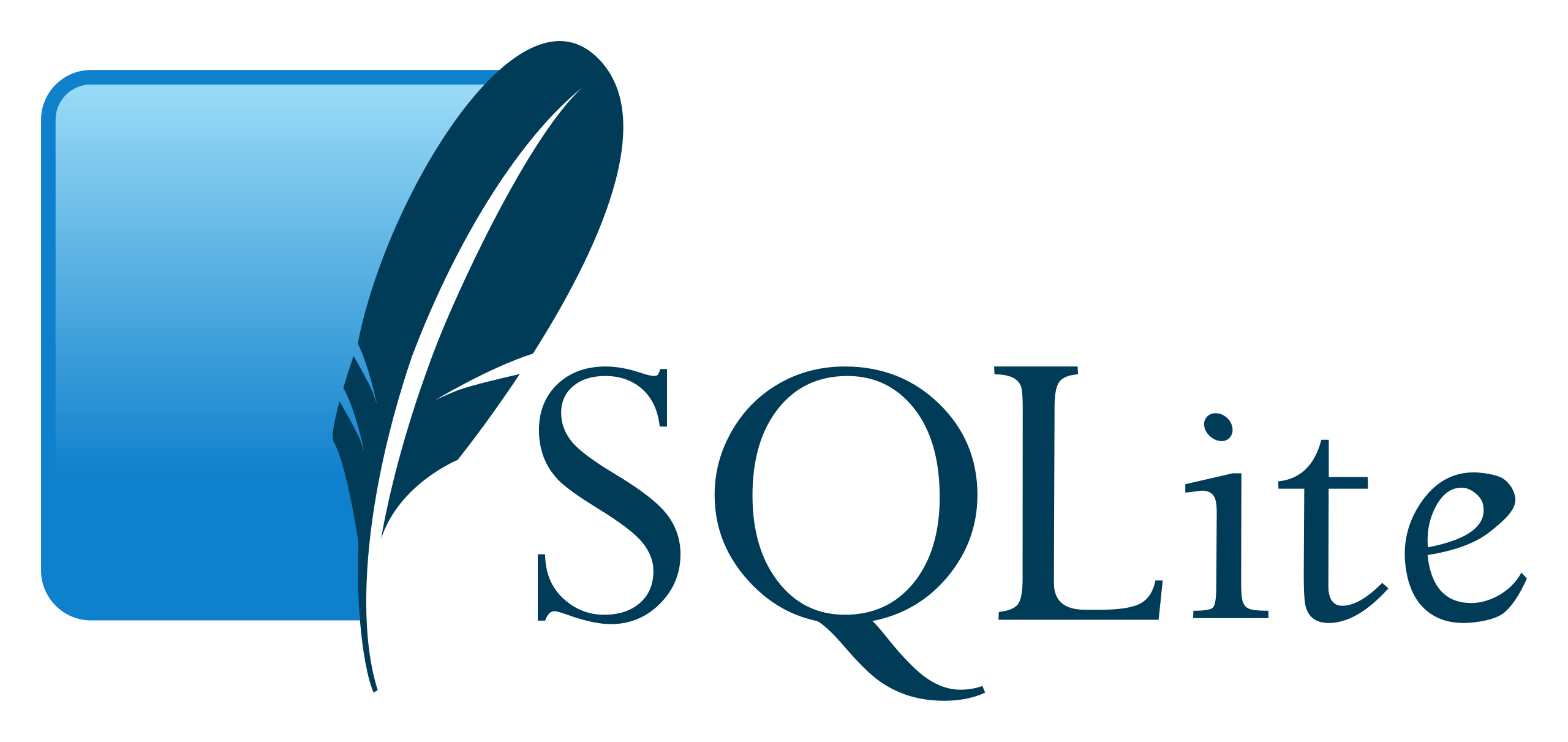
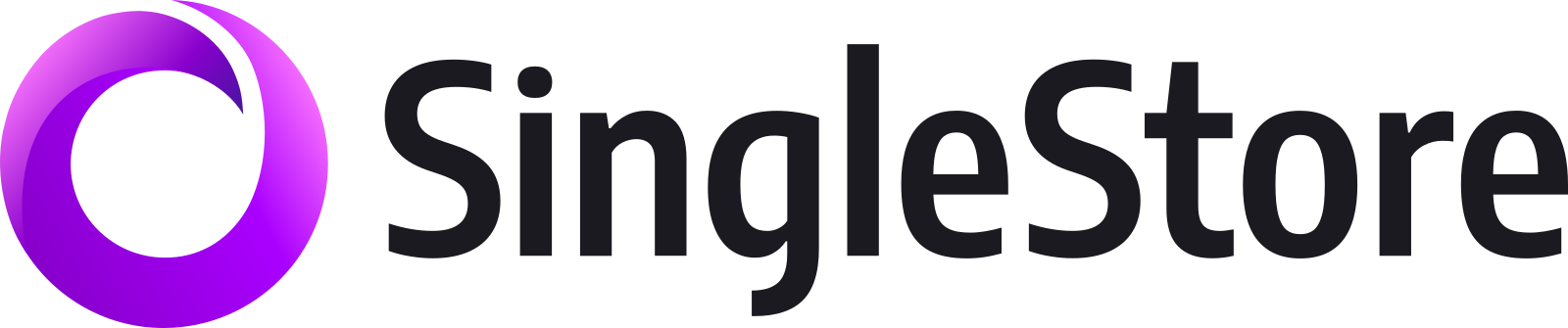
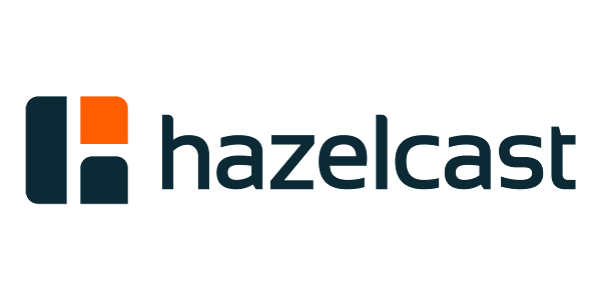
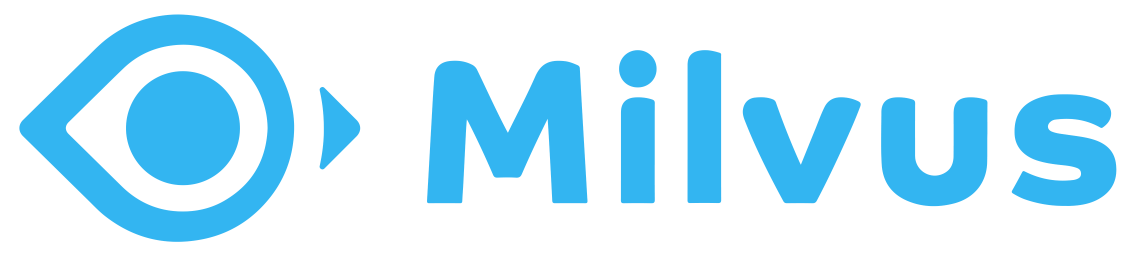
[GET STARTED]
START SERVING IN SECONDS
from feast import FeatureStore
# Initialize the feature store
store = FeatureStore(repo_path="feature_repo")
# Get features for training
training_df = store.get_historical_features(
entity_df=training_entities,
features=[
"customer_stats:daily_transactions",
"customer_stats:lifetime_value",
"product_features:price"
]
).to_df()
# Get online features for inference
features = store.get_online_features(
features=[
"customer_stats:daily_transactions",
"customer_stats:lifetime_value",
"product_features:price"
],
entity_rows=[{"customer_id": "C123", "product_id": "P456"}]
).to_dict()
# Retrieve your documents using vector similarity search for RAG
features = store.retrieve_online_documents(
features=[
"corpus:document_id",
"corpus:chunk_id",
"corpus:chunk_text",
"corpus:chunk_embedding",
],
query="What is the biggest city in the USA?"
).to_dict()
[BLOG POSTS]
THE LATEST FROM FEAST
Retrieval Augmented Generation with Feast
How Feast empowers ML Engineers to ship RAG applications to Production.
The Road to Feast 1.0
Exploring the goals and vision for Feast 1.0, including tighter Kubeflow integration, enterprise features, and graduation from LF AI and Data Foundation Incubation.
The Future of Feast
A look at Feast's journey, its evolution as a feature store, and the exciting path ahead with new maintainers and community-driven development.
[GET STARTED]
START BUILDING TODAY
Join our Slack
Become part of our developer community & get support from the Feast developers
Join Community